18-过渡动画
码路教育 6/3/2022
# 1, Vue中的动画
# 1.1, Vue中的动画介绍
Vue中为我们提供一些内置组件和对应的API来完成动画,利用它们我们可以方便的实现过渡动画效果
- 没有动画的情况下,整个内容的显示和隐藏会非常的生硬
- 如果我们希望给单元素或者组件实现过渡动画,可以使用 transition 内置组件来完成动画
# 1.2, Vue的transition动画
Vue 提供了 transition 的封装组件,在下列情形中,可以给任何元素和组件添加进入/离开过渡
- 条件渲染 (使用 v-if)条件展示 (使用 v-show)
- 动态组件
- 组件根节点
当插入或删除包含在 transition 组件中的元素时,Vue 将会做以下处理
- 自动嗅探目标元素是否应用了CSS过渡或者动画,如果有,那么在恰当的时机添加/删除 CSS类名
- 如果 transition 组件提供了JavaScript钩子函数,这些钩子函数将在恰当的时机被调用
- 如果没有找到JavaScript钩子并且也没有检测到CSS过渡/动画,DOM插入、删除操作将会立即执行
过渡动画class(Vue就是帮助我们在这些class之间来回切换完成的动画)
- v-enter-from:定义进入过渡的开始状态
- 在元素被插入之前生效,在元素被插入之后的下一帧移除。
- v-enter-active:定义进入过渡生效时的状态,
- 在整个进入过渡的阶段中应用,在元素被插入之前生效,在过渡/动画完成之后移除。这个类可以被用来定义进入过渡的过程时间,延迟和曲线 函数
- v-enter-to:定义进入过渡的结束状态
- 在元素被插入之后下一帧生效 (与此同时 v-enter-from 被移除),在过渡/动画完成之后移除
- v-leave-from:定义离开过渡的开始状态
- 在离开过渡被触发时立刻生效,下一帧被移除
- v-leave-active:定义离开过渡生效时的状态
- 在整个离开过渡的阶段中应用,在离开过渡被触发时立刻生效,在过渡/动画完成之后移除。这个类可以被用来定义离开过渡的过程时间,延迟 和曲线函数
- v-leave-to:离开过渡的结束状态
- 在离开过渡被触发之后下一帧生效 (与此同时 v-leave-from 被删除),在过渡/动画完成之后移除
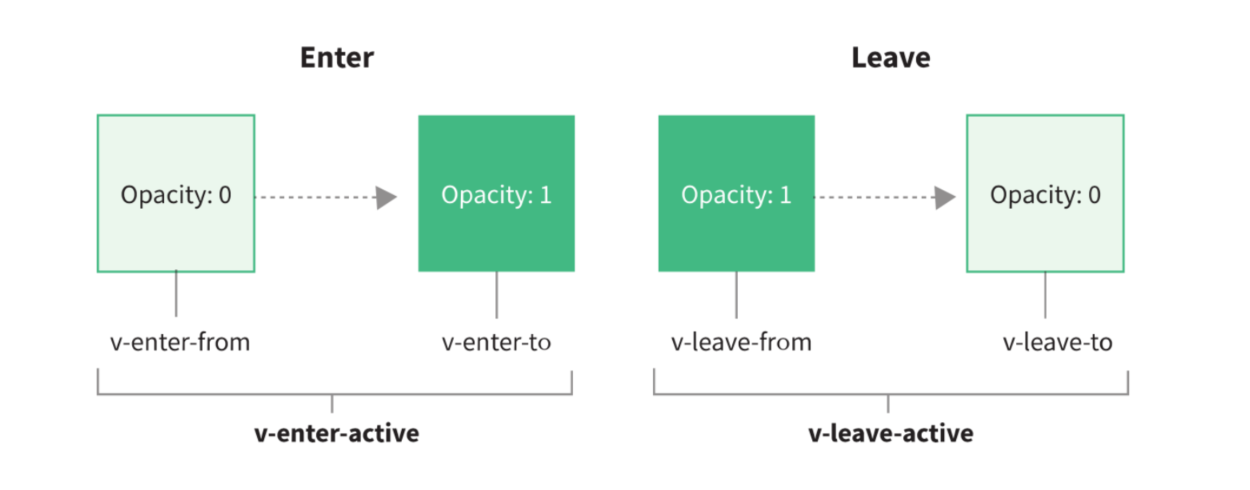
class的name命名规则如下
- 如果我们使用的是一个没有name的transition,那么所有的class是以 v- 作为默认前缀
- 如果我们添加了一个name属性,比如
,那么所有的class会以 wc- 开头
// App.vue
<template>
<div>
<button @click="show = !show">点击我切换显示与隐藏</button>
<!-- transiton:组件,全局组件。可以在任意组件内直接使用-->
<!-- name属性值:如果页面中有多个多度动画需求,区分样式类名。 如果没有书写name,默认类名v-xxx开头,
如果有name属性值,类名就以name属性值开头使用。这样可以去多个过渡动画效果!!!
-->
<transition name="jch">
<div class="box" v-show="show">
<h3>我是H3标题</h3>
</div>
</transition>
</div>
</template>
<script>
export default {
name: "",
data() {
return {
show: false,
};
},
};
</script>
<!-- 在style标签身上加上一个lang=less属性,代表style里面书写的less样式 -->
<!-- 进入阶段的过渡动画 -->
<style scoped lang="less">
@color: cyan;
@f: red;
.box {
width: 400px;
height: 200px;
background: @color;
h3 {
color: @f;
}
}
/* 进入阶段的过渡动画*/
/*进入开始阶段*/
.jch-enter {
opacity: 0;
transform: rotate(0deg);
}
/* 定义进入阶段过渡动画时间、速率类名地方*/
.jch-enter-active {
/*定义过渡动画时间、速率等等*/
transition: all 5s;
}
/*进入结束阶段*/
.jch-enter-to {
opacity: 1;
transform: rotate(360deg);
}
/*定义离开阶段的过渡动画*/
/*
离开阶段的开始状态
*/
.jch-leave {
opacity: 1;
transform: scale(1);
}
/* 定义离开阶段过渡动画时间、速率类名地方*/
.jch-leave-active {
transition: all 3s;
}
/*离开阶段的结束状态*/
.jch-leave-to {
opacity: 0;
transform: scale(0);
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
过渡动画遮罩层
// components/ElDialog.vue
<template>
<div>
<transition>
<div class="mask" v-show="visible"></div>
</transition>
</div>
</template>
<script>
export default {
name: "",
props: ["visible"],
};
</script>
<style scoped>
.mask {
position: fixed;
left: 20%;
top: 20%;
right: 20%;
bottom: 20%;
background: rgba(0, 0, 0, 0.5);
z-index: 1000;
}
.v-enter {
transform: scale(0);
}
.v-enter-active {
transition: all 2s;
}
.v-enter-to {
transform: scale(1);
}
.v-leave{
transform: scale(1);
border-radius: 0px;
}
.v-leave-active{
transition: all 2s;
}
.v-leave-to{
transform: scale(0);
border-radius:50%;
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
// App.vue
<template>
<div>
<button @click="visible=!visible">我是按钮需要遮罩层组件</button>
<ElDialog :visible="visible"></ElDialog>
</div>
</template>
<script>
import ElDialog from '@/components/ElDialog'
export default {
name: '',
data() {
return {
visible:false
}
},
components:{
ElDialog
}
}
</script>
<style scoped>
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26