02-属性节点操作
码路教育 7/19/2022
# 1. 元素的属性之attribute
一个元素除了有开始标签、结束标签、内容之外,还有很多的属性(attribute)
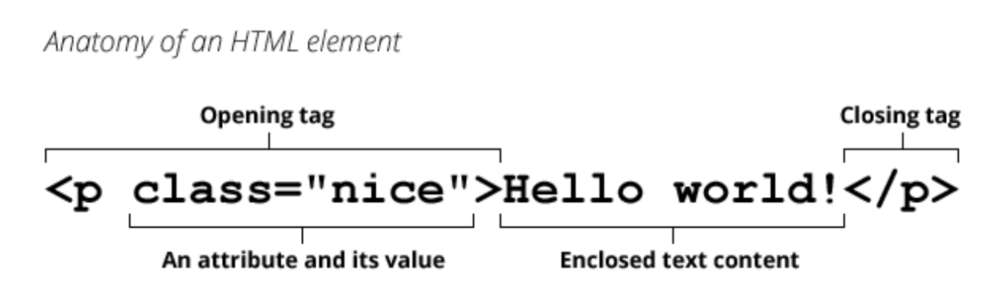
浏览器在解析HTML元素时,会将对应的attribute也创建出来放到对应的元素对象上。
- 比如id、class就是全局的attribute,会有对应的id、class属性;
- 比如href属性是针对a元素的,type、value属性是针对input元素的;
attribute的分类
- 标准的attribute:某些attribute属性是标准的,比如id、class、href、type、value等;
- 非标准的attribute:某些attribute属性是自定义的,比如abc、age、height等;
# 2. attribute的操作
对于所有的attribute访问都支持如下的方法:
- elem.hasAttribute(name) — 检查特性是否存在。
- elem.getAttribute(name) — 获取这个特性值。
- elem.setAttribute(name, value) — 设置这个特性值。
- elem.removeAttribute(name) — 移除这个特性。
- attributes:attr对象的集合,具有name、value属性;
attribute具备以下特征:
- 它们的名字是大小写不敏感的(id 与 ID 相同)。
- 它们的值总是字符串类型的。
<body>
<div class="father" title="hehe" score="88" id="box">
<div class="son" abc="abc" age="18">
son
</div>
</div>
<script>
let boxEle = document.querySelector("#box");
// 可以判断标准的、也可以判断非标准的
// console.log(boxEle.hasAttribute("class"));
// console.log(boxEle.hasAttribute("title"));
// console.log(boxEle.hasAttribute("name"));
// console.log(boxEle.hasAttribute("score"));
// 通过Attribute的属性名,获取属性值
// console.log(boxEle.getAttribute("class"));
// 设置Attribute
// boxEle.setAttribute("adress","bj")
// 删除Attribute
// boxEle.removeAttribute("title")
// 得到所有的Attribute
console.log(boxEle.attributes);
</script>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
# 3. 元素的属性之property(打点形式)
对于标准的attribute,会在DOM对象上创建与其对应的property属性:
在大多数情况下,它们是相互作用的
- 改变property,通过attribute获取的值,会随着改变;
- 通过attribute操作修改,property的值会随着改变;(但是input的value修改只能通过attribute的方法)
除非特别情况,大多数情况下,设置、获取attribute,推荐使用property的方式
- 因为它默认情况下是有类型的;
<body>
<div class="father" title="hehe" score="88" id="box">
<div class="son" abc="abc" age="18">
son
</div>
</div>
<input type="text" class="ipt" value="我是默认值">
<hr>
<!-- checked="checked" -->
<input type="checkbox" class="ipt2">
<script>
let boxEle = document.querySelector("#box");
let iptEle = document.querySelector(".ipt")
let iptEle2 = document.querySelector(".ipt2")
// obj是对象 对象是属性(property)的无序集合
// 所谓的property就是对象的中的属性
// let obj = {
// name:"wc",
// age:18
// }
// boxEle.setAttribute("title","xixi"); // 改变了attribute
// console.log(boxEle.title);
// boxEle.title = "heihei"; // 改变了propety
// console.log(boxEle.getAttribute("title"));
// iptEle.setAttribute("value","lala")
// iptEle.value = "hehe"
// 建议使用property
console.log(iptEle2.checked);
console.log(iptEle2.getAttribute("checked"));
</script>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
<body>
<div class="father" id="abc" title="hehe" age="18" score="88">
我是一个DVI
</div>
<!-- <input type="checkbox" checked="checked"> -->
<input type="checkbox" checked>
<hr>
<input type="text" class="account">
<button class="btn">设置input的值</button>
<script>
let divEle = document.getElementById("abc");
console.log(divEle.getAttribute("id"));
console.log(divEle.getAttribute("age"));
console.log("-----------------");
console.log(divEle.id);
// 对于非标准的attribute 是没有 对应的property
console.log(divEle.age); // undefined
console.log("-------------------");
let iptEle = document.getElementsByTagName("input")[0];
// if(iptEle.getAttribute("checked")){
// console.log("处于选中状态");
// }
if(iptEle.checked){
console.log("处于选中状态");
}
console.log(typeof iptEle.checked);
console.log("-------------------");
let iptEle2 = document.getElementsByTagName("input")[1];
let btnEle = document.getElementsByClassName("btn")[0];
btnEle.onclick = function(){
// 通过property设置数据时,优先级是高于attribute
iptEle2.value = "66666"; // 通过property来设置
iptEle2.setAttribute("value",88888); // 通过attribute来设置
}
</script>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
# 4. HTML5的data-*自定义属性
*HTML5的data-自定义属性,它们可以在dataset属性中获取到的
<body>
<!-- 以data-打头的,叫html5中的自定义属性 -->
<div class="father" age="18" data-score="88" data-address="bj">
我是一个孤独的DIV
</div>
<script>
let boxEle = document.querySelector(".father");
console.log(boxEle.dataset);
console.log(boxEle.dataset.score);
console.log(boxEle.dataset.address);
</script>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12