13-CSS3
码路教育 6/20/2022
# 一、CSS3中的处理盒子
# 01. 盒子阴影
<head>
<style>
div {
width: 100px;
height: 100px;
background: red;
margin: 30px;
color: #fff;
float: left;
}
div:nth-child(1) {
box-shadow: 20px 0 black;
}
div:nth-child(2) {
box-shadow: 0px -20px black;
}
div:nth-child(3) {
box-shadow: 20px 20px black;
}
div:nth-child(4) {
box-shadow: -20px -20px black;
}
div:nth-child(5) {
box-shadow: 0 0 20px black;
}
div:nth-child(6) {
box-shadow: 0 0 20px black inset;
}
div:nth-child(7) {
box-shadow: 10px 10px 20px black, -10px -10px 20px green;
}
</style>
</head>
<body>
<div> 水平方向的阴影</div>
<div> 垂直方向的阴影 </div>
<div> 右下角的阴影</div>
<div> 左上角的阴影 </div>
<div> 阴影扩散</div>
<div> 内部发光</div>
<div> 多重阴影</div>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52

# 02. 文字阴影
<head>
<style>
p {
width: 800px;
height: 300px;
font-size: 70px;
background-color: orange;
line-height: 300px;
text-align: center;
/* 文字阴影 */
/* text-shadow: 10px 10px 10px yellow; */
color: orange;
/* 突起效果 */
/* text-shadow: -1px -1px 0px white,1px 1px 0 #000; */
/* 凹陷效果 */
text-shadow: -1px -1px 0px black, 1px 1px 0 white;
}
</style>
</head>
<body>
<p>这是一段有阴影的文字</p>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
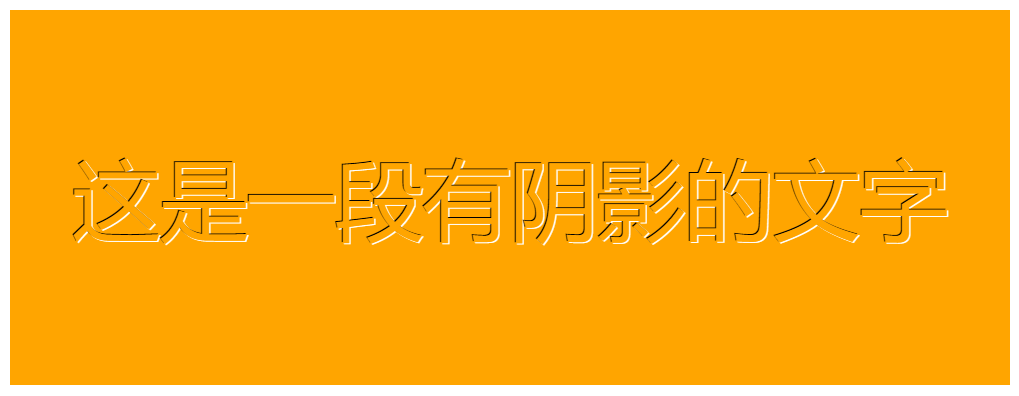
# 03. 背景
<head>
<style>
div {
/* 背景颜色 */
background-color: aqua;
/* 背景图片 */
background-image: url(./images/01.jpg);
/* 平铺 */
background-repeat: no-repeat;
/* 定位 */
background-position: 10px;
/* 背景大小 */
background-size: auto;
/* 混合写法 */
background: red url(./images/01.jpg) no-repeat 10px 10px;
}
</style>
</head>
<body>
<div></div>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
# 03. 背景裁剪
<head>
<style>
div {
width: 300px;
height: 300px;
background: url(./images/15.jpg) no-repeat;
border: 1px dashed red;
padding: 50px;
background-size: 100%;
/* 裁剪 */
/* 把边框下面的去掉 */
/* background-clip: padding-box; */
/* 把我们设置的内边距去掉 */
background-clip: content-box;
}
</style>
</head>
<body>
<div></div>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
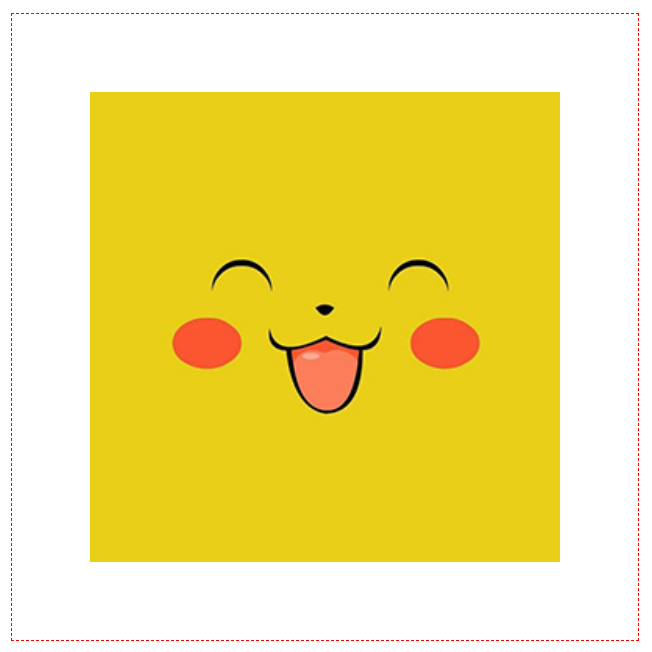
# 05. 渐变背景
<head>
<style>
div {
width: 100px;
height: 100px;
margin: 30px;
border: 1px solid red;
float: left;
}
/* 渐变属性 */
/* 默认角度 180deg 起始色 结束色 */
/* 角度 deg */
div:nth-child(1) {
background: linear-gradient(90deg, red, orange, yellow, green, blue, skyblue, purple);
}
div:nth-child(2) {
background: linear-gradient(0deg, red, orange, yellow, green, blue, skyblue, purple);
}
div:nth-child(3) {
background: linear-gradient(270deg, red, orange, yellow, green, blue, skyblue, purple);
}
div:nth-child(4) {
background: linear-gradient(45deg, red, orange, yellow, green, blue, skyblue, purple);
}
div:nth-child(5) {
background: linear-gradient(225deg, red, orange, yellow, green, blue, skyblue, purple);
}
</style>
</head>
<body>
<div>从左到右</div>
<div>从下到上</div>
<div>从右到左</div>
<div>从左下到右上</div>
<div>从右下到左上</div>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
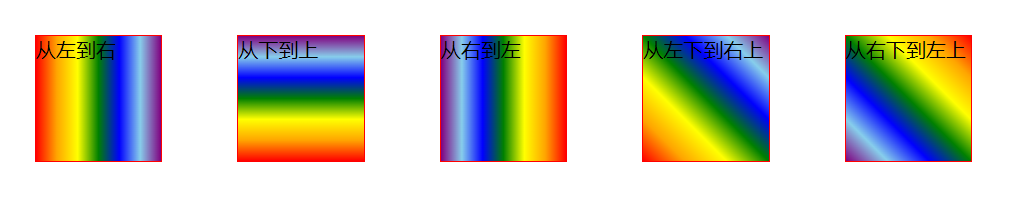
# 06. 径向渐变
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 200px;
height: 200px;
border: 1px solid red;
/* 径向渐变 radial-gradient */
/* 参数1:X轴
参数2:Y轴 */
background: radial-gradient(200px 100px, red, yellow, blue);
}
</style>
</head>
<body>
<div></div>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
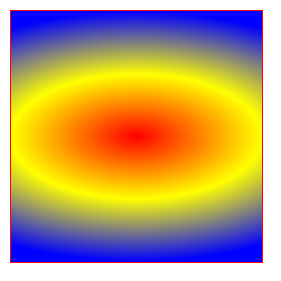
# 07. 透明度
<head>
<style>
div {
width: 200px;
height: 200px;
margin-top: 20px;
background-color: red;
}
div:nth-child(1) {
/* 子元素不继承透明度,只对已经设置的盒子起效 */
background-color: rgba(255, 0, 0, .5);
}
div:nth-child(2) {
/* 子元素继承透明度 */
opacity: 0.5;
}
div:nth-child(3) {
/* 表示全透明 */
background-color: transparent;
}
.son {
width: 50px;
height: 50px;
background-color: yellow !important;
}
</style>
</head>
<body>
<!-- 透明度
1.rgba
2.opacity
3.transparent (透明) -->
<div>
<div class="son">我是son</div>
</div>
<div>
<div class="son">我是son</div>
</div>
<div>
<div class="son">我是son</div>
</div>
</body>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47